Getting Started with Python for Beginners #10: Putting It All Together – A Small Project
Congratulations! You’ve reached the final post in our “Getting Started with Python for Beginners” series. So far, we’ve covered a wide range of foundational topics:
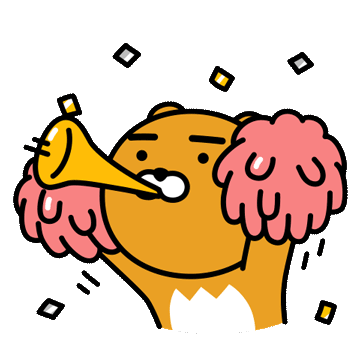
- Installing Python and setting up your environment
- Working with variables, data types, and control flow
- Using lists, tuples, and dictionaries to manage data
- Writing functions and understanding object-oriented programming concepts
- Handling files, modules, and libraries
- Debugging your code and managing errors gracefully
Now it’s time to put everything you’ve learned into practice by building a small, command-line project. This project will bring together these concepts and show you how they can form the backbone of a real application.
Project Idea: A Simple Quiz Game
Let’s create a quiz game application. This will allow us to practice:
- Data Handling: Storing questions and answers.
- Control Flow: Deciding how to handle correct and incorrect answers.
- Functions: Organizing code for asking questions and evaluating answers.
- Files: Reading questions from a file.
- OOP (optional): Representing questions as objects for clarity.
- Error Handling: Gracefully dealing with unexpected input.
What the app will do:
- Load multiple-choice questions from a file.
- Present each question to the player.
- Allow the player to select an answer.
- Keep score and display the final result.
Step-by-Step Outline
- Data Preparation:
Create a file named questions.txt that contains questions, options, and the correct answer. For example:Each block has a question, four options, and the correct answer on a separate line. A blank line separates each question block. - What is the capital of France? A) Berlin B) Madrid C) Paris D) Rome C What does CPU stand for? A) Central Processing Unit B) Computer Personal Unit C) Central Personal Unit D) Control Processing Unit A
- Question Class (Optional):
Define a Question class to store a single question, its options, and the correct answer.While OOP isn’t strictly necessary, it helps keep data structured and makes the code more readable. - class Question: def __init__(self, prompt, options, answer): self.prompt = prompt self.options = options self.answer = answer
- Loading Questions from a File:
Write a function to read questions.txt and return a list of Question objects.Here, we:- Read all the file content.
- Split it into question blocks.
- For each block, the first line is the question, the next four lines are options, and the sixth line is the correct answer.
- def load_questions(filename): questions = [] with open(filename, "r", encoding="utf-8") as file: content = file.read().strip() blocks = content.split("\n\n") # Split by blank lines for block in blocks: lines = block.split("\n") prompt = lines[0] options = lines[1:5] # A, B, C, D lines answer = lines[5].strip() questions.append(Question(prompt, options, answer)) return questions
- Asking Questions and Getting User Input:This function prints the question and options, then loops until the user enters a valid choice.
- def ask_question(question): print("\n" + question.prompt) for opt in question.options: print(opt) while True: user_input = input("Your answer (A/B/C/D): ").upper().strip() if user_input in ["A", "B", "C", "D"]: return user_input else: print("Invalid choice. Please enter A, B, C, or D.")
- Main Quiz Logic:This function iterates through each question, asks the user for an answer, compares it to the correct answer, updates the score, and displays the final tally.
- def run_quiz(questions): score = 0 for q in questions: user_answer = ask_question(q) if user_answer == q.answer: print("Correct!") score += 1 else: print(f"Incorrect! The correct answer is {q.answer}.") print(f"\nYou got {score} out of {len(questions)} questions correct!")
- Error Handling:
- If the file questions.txt is missing or in the wrong format, we can wrap the loading logic in a try-except block.
- If no questions are loaded, print a friendly error message.
def main(): try: questions = load_questions("questions.txt") if not questions: print("No questions found. Please check the file format.") return run_quiz(questions) except FileNotFoundError: print("The file 'questions.txt' was not found.") except Exception as e: print("An unexpected error occurred:", e) if __name__ == "__main__": main()
Testing the Application
- Check for correct answers: Ensure that when you choose the correct answer, it increments the score.
- Check for incorrect answers: Make sure it shows the correct answer when you’re wrong.
- Invalid inputs: If you type something other than A-D, the program should ask again instead of crashing.
- Missing file: Temporarily rename or remove questions.txt and see if it shows the error message.
Expanding the Project
Feel free to enhance this quiz game:
- Add more question types: True/False questions, fill-in-the-blank, or multiple correct answers.
- Randomize questions: Use the random module to shuffle the question list.
- Track high scores: Store the user’s best score in a file.
- Use OOP more extensively: Create a Quiz class to manage the whole process.
Reviewing Key Concepts
This project integrated many concepts:
- Variables and data types: Storing questions and answers.
- Control flow: Repeating questions and checking user input.
- Functions: ask_question(), run_quiz(), and load_questions() clearly define tasks.
- Files: Reading questions from a text file.
- OOP: Defining a Question class.
- Error handling: Using try-except to handle missing files.
- Testing and debugging: Ensuring the program behaves correctly under various conditions.
By combining these elements, you’ve built a complete, if simple, application in Python.
Wrapping Up
You’ve come a long way since the start of this series. You’ve gone from installing Python to building a functional application that integrates multiple programming concepts. This is just the beginning of your Python journey!
As a next step, consider exploring more advanced topics based on your interests:
- Web development: Check out frameworks like Django or Flask.
- Data science and machine learning: Explore libraries like NumPy, Pandas, and scikit-learn.
- Game development: Try Pygame for building simple 2D games.
- Automation and scripting: Use Python to automate repetitive tasks on your computer.
The possibilities are endless. Keep learning, keep experimenting, and have fun programming!